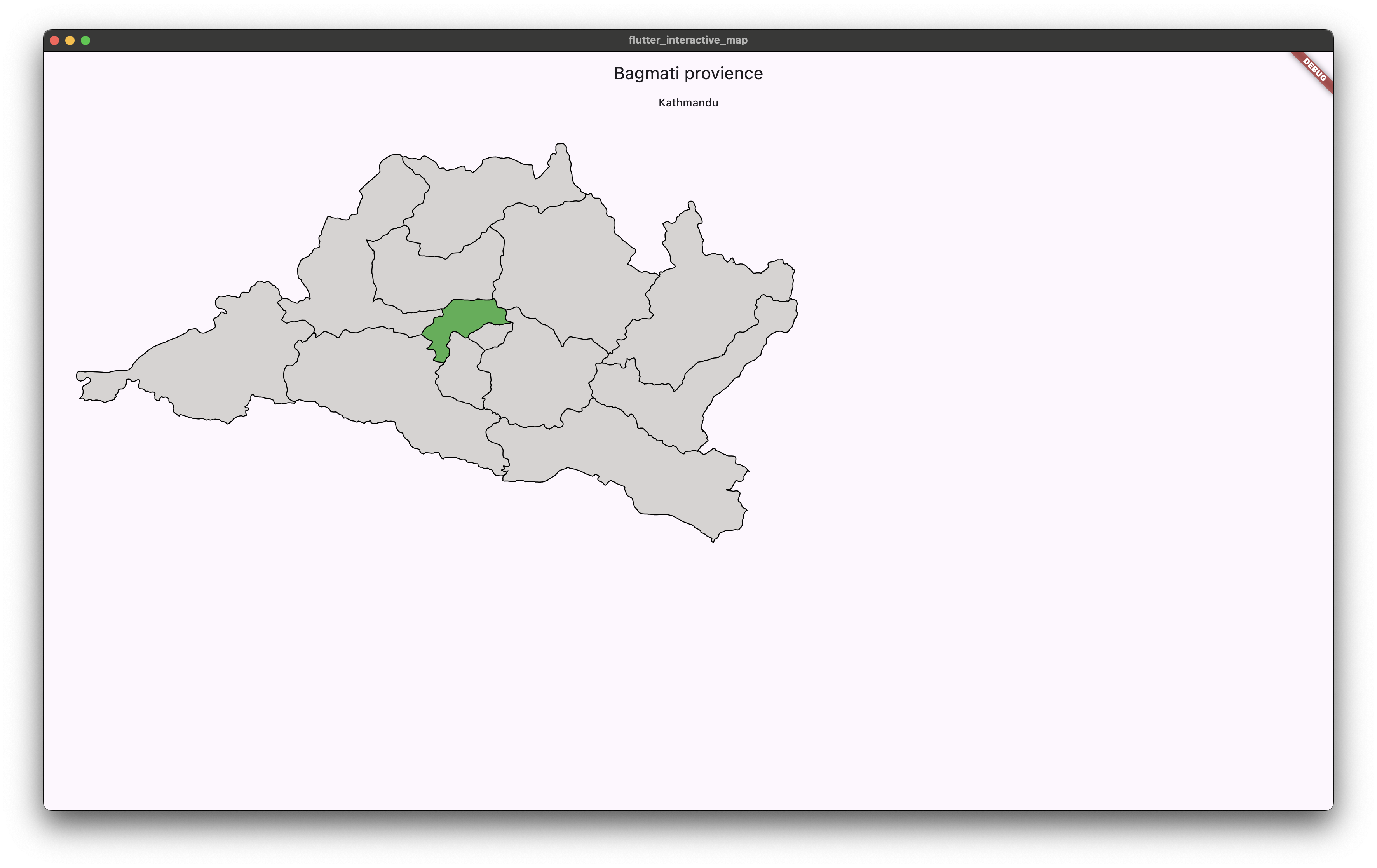
Blog category: Flutter
Use SVG to build interactive maps in Flutter. This can be used for various purposes including games, educational apps, and more.
Google generative AI with Flutter using the official Dart SDK
Use the official Dart SDK to access Google's generative AI models in Flutter and build badass apps powered by Gemini.
Dart mixins for everyone
Mixins in Dart are a way of reusing a class's code in multiple class hierarchies. Mixins can be added to different classes to extend their capabilities, without the need for complex inheritance structures.
Asynchronous programming with streams in Dart and Flutter
Learn how to leverage Streams, StreamController, and StreamBuilder in Dart and Flutter for efficient asynchronous programming. Explore code examples and step-by-step explanations to master working with streams and building dynamic widgets.
Achiving effective separation of UI and business logic in Flutter
Learn the most valuable tip in Flutter to maintain a clear separation between UI and business logic. Enhance codebase, improve development process, and enjoy benefits like modularity, collaboration, and better code organization.
Using the url_launcher Plugin in Flutter - A Comprehensive Guide
Explore our comprehensive guide on using the url_launcher plugin in Flutter. Learn how to install it, launch various types of URLs including web, phone call, SMS, and email, plus its pros, cons, and alternatives. Perfect for Flutter developers!
A Beginner's Guide to Persisting Data with Shared Preferences in Flutter
Explore how to use the shared_preferences plugin in Flutter for local data storage. Learn the ins and outs of saving, reading, and removing data for a smooth user experience.
A Beginner's Guide to the HTTP Package in Flutter
Learn to leverage the power of Flutter's http package for your app development needs. Our guide covers everything from basic GET and POST requests to advanced error handling and interceptors. Ideal for beginners, it includes comprehensive code examples for a hands-on learning experience.
Diving Deeper into Asynchronous Programming with Futures in Flutter
Boost your Flutter skills with this comprehensive guide on asynchronous programming. Learn about Dart Futures, chaining, FutureBuilder, and error handling with real-world examples.
Making Friends with Asynchronous Programming and Futures in Flutter
Unleash the power of Asynchronous Programming in your Flutter apps. Learn how to implement Futures and handle errors for a seamless user experience.
The #30DaysMasterFlutter Journey Has Begun! 🚀
We're thrilled to announce that the journey to mastering Flutter and Dart begins today with our #30DaysMasterFlutter course! This free, easy to follow, project-based journey 📚 is your ticket to learning one of the most exciting technologies in the world of app development.
Get Started with Testing in Flutter
Testing is a crucial part of the software development process. Writing and running automated tests make sure our application remains robust with every change.
Let's Build an Android Launcher Application with Flutter
Launcher app is one of the most important application for android mobile users. In this article we are going to learn to build Android launcher application using Flutter framework.
Recommended Flutter Plugins and Packages for Every Project
What makes Flutter great are it's ecosystem of packages and plugins that make it possible to build complex integrations and functionalities in a fraction of seconds. Lets look at some of the plugins and plackages that are recommended for every Flutter project you create.
Demystifying Dart Extension Methods
Dart extension methods provide us with a easy and clean way to extend external libraies to our specific needs without touching their source code. Let's learn what they are and how to use them.
Integrating with REST API in Flutter
In this article we will introduce REST API briefly and then learn to integrate external service into our Flutter application using REST API. We will be using the official http package however after learning the fundamentals you will be able to use any other library to make a http request in Flutter.
Flutter Calendar App Optimizing Query and Managing Events
In previous two parts we learned to setup calendar and load and display events from Firestore. In this third and final part in the series, we will optimize the Firestore query so that we will only load the events necessary for the visible days in the calendar. Then we will also setup CRUD to allow to add, update and delete the events.
Loading and Displaying Events from Firestore in Flutter Calendar App
In this second part of Flutter Calendar App tutorial, learn to integrate Firebase and then load and display event markers in calendar from Firestore database.
Building Calendar App with Flutter
Calendar is integral to various types of applications. From a simple scheduling application to complex management systems, calendar plays important role. In this tutorial we will learn to display and customize a calendar in our Flutter application.